In this coure, we will learn to use Promise in the JavaScript. Before we start, make sure to subscribe to CRM Crate so that you stay up-to-date in field of Microsoft Dynamics 365 CRM.
What is Promise in the JavaScript?
Promise in JavaScript is used to architect an asynchronous code in a synchronous way. In simple words, the Promises are asynchronous callbacks which are much more readable in terms of error handling.
Why should we use Promise in JavaScript?
Consider a real-time scenario where a JavaScript has two functions namely “Apple” and”Mango”. In the exeuction cycle, the function “Apple” takes significantly more time to execute than the function “Mango”. Also the script in function “Mango” depends upon the data returned as a result from the function “Apple”.

With help of “Promise”, we can bind both these functions together in such a way that when the Promise makes result available to function “Mango” when it is ready from the function “Apple”.
In some words, the implementation of Promise can make the execution cycle wait till the result is available from the promise implemented function.
Core fundamental structure of Promise
Below is the fundamentals of promise .
var promise = new Promise(function (resolve, reject) { let condition = 1; if (condition < 1) resolve(); else reject(); });
The function passed to the Promise is called as “Executor”. When a promise is executed, it contains a producable logic code which will eventually produce a result. As soon as the Executor obtains the result whether soon or late, it calls one of the callback –
- resolve(value) — If the execution is finished successfully.
- reject(error) — If an error occurred.
JavaScript Promise example in Dynamics 365 CRM
In the below example, we have implemented the promise in the function which is calling an asynchronous action such as a web service request.
//CRM Crate - Promise Implementation.
function ExecutionMethod(executionContext) {
//Input Parameter For Our Web Service
var parameters = {
InputParam: "Which is the best CRM?"
};
var webserviceData = new actionProcessingMethod(parameters.InputParam);
try {
//Step 1 - Call The Promise Method.
var PromiseReturn = ImplementPromise(webserviceData, executionContext);
//Step 2 - Bind The Promise Function.
PromiseReturn.then(function (response) {
if (response != null) {
//Display The Promise Output From Async Web Service.
console.log("Webservice Output Parameter : " + response);
}
});
}
catch (e) {
console.log("Error Occured in while calling process : " + JSON.stringify(e));
}
};
var ImplementPromise = function (actionData, executionContext) {
//Step 3 - Initiate The Promise Function.
return new Promise(function (resolve) {
//Step 4 - Call The Async Web Service.
Xrm.WebApi.online.execute(actionData).then(
function (data) {
if (data.ok) {
data.json().then(function (response) {
//Step 5 - Resolve The Promise On Valid Webservice Response.
if (response.OutputParam != null) {
PromiseOutput = response.OutputParam;
resolve(PromiseOutput);
}
else {
resolve(false);
console.log("Error Occured While Calling Web Service : " + response.SuccessCallBack);
}
});
}
},
function (error) { alert(error.message || error.description); }
);
});
};
//Method To Process Our Web Service.
var actionProcessingMethod = function (InputParam) {
this.InputParam = InputParam;
this.getMetadata = function () {
return {
boundParameter: null,
parameterTypes: {
"InputParam": {
"typeName": "Edm.String",
"structuralProperty": 1
}
},
operationType: 0,
operationName: "cc_DemoAction"
};
};
};
Code Explaination –
- Step 1 – Call the function where the Promise is been implemented.
- Step 2 – Bind the current function with the promise function so that it awaits till the promise function provides the result.
- Step 3 – Initiate the Promise function.
- Step 4 – Call the asynchronous webservice within the Promise function.
- Step 5 – Resolve the Promise on the success event of the webservice.
Validating the JavaScript snippet in real-time console –
- Below we have called the Promise function –

- Once the called Promise function yeilds webservice response then we have resolved the promise –

- The second dependent function is been called only after the main promise function is completely executed –
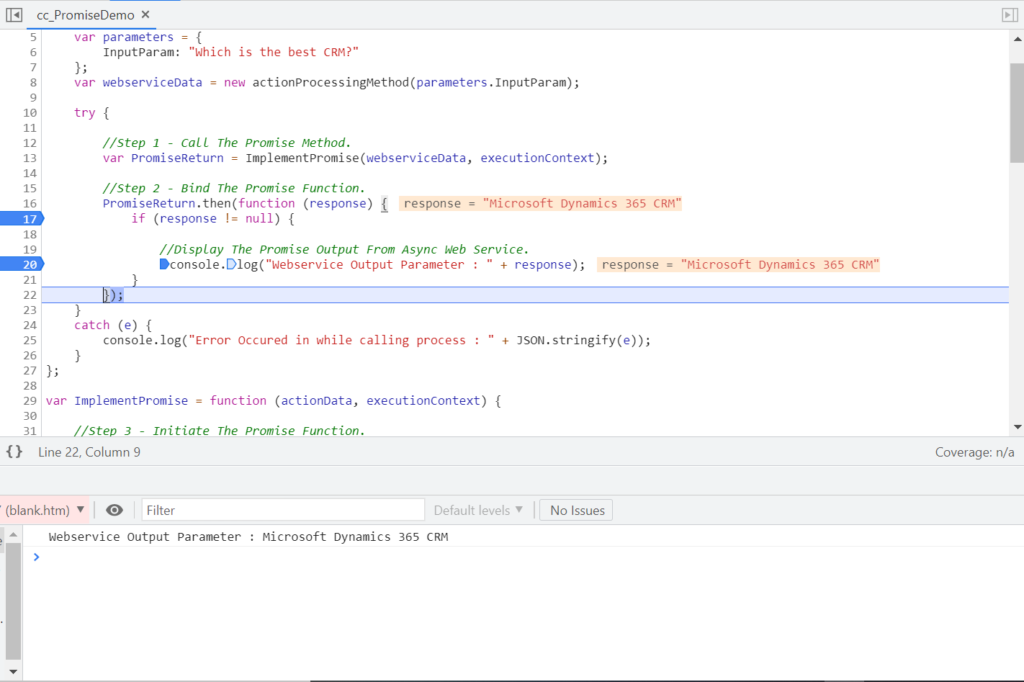
Thus we leanred to implement and use the JavaScript Promise in Dynamics 365 CRM.
CRM Crate
All In One Platform For Learning Microsoft CRM.
[…] We will call a custom action during the OnSave event of an entity. Since calling a custom action (Web Service) is an aynschronous process, we will use the promise for awaiting the action response. Click here to learn more Promise in JavaScript. […]