We will learn to Get & Set value in an Environment Variable of Dynamics 365. Before we start, make sure to subscribe to CRM Crate so that you can stay up to date in the field of Dynamics 365 / Power Apps.
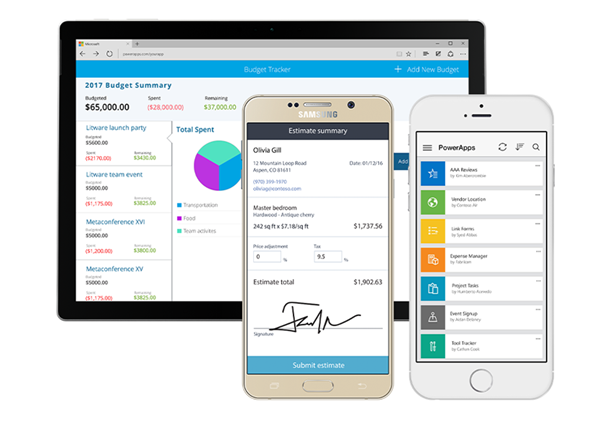
What is an Environment Variable?
Environment variables are used to store the parameter keys and values, which then serve as input to various other application objects. We can use the Environment Variables to store critical data such as credentials, service keys and other important information required in the Dynamics 365.
Below are the benefits of using environmental variables –
- Store the configuration related information for the data sources used in Power Apps and Power Automate flows.
- One environment variable can be used across many different solution components – whether they’re the same type of component or different.
- Provide new parameter values while importing solutions to other environments.
- Package and transport your customization and configuration together and manage them in a single location.
- Package and transport secrets, such as credentials used by different components, separately from the components that use them.
- Additionally, if you need to retire a data source in production environments, you can simply update the environment variable values with information for the new data source.
- The environment variables can be unpacked and stored in source control. You may also store different environment variables values files for the separate configuration needed in different environments.
How to create an Environment Variable?
Follow the below steps for creating an environmental variable in Dynamics 365 / Power Apps.
Step 1 – Open Power Apps studio and navigate to the solution as per your requirement.

Step 2 – In the solution explorer, navigate to Environment Variables as shown below.

Step 3 – Configure the variable as per your requirement.

How to retrieve & update an Environment Variable using JavaScript?
In our scenario, we have first retrieved an existing value from our variable and later collected the new value from Dynamics 365 record form, updated this newly collected value in our environment variable. The below JavaScript snippet is triggered during the OnChange event of form fields.
//Main Method For Getting Variable
async function EnvironmentalVariableExecutionGet (executionContext) {
//Create a Form Context.
var formContext = executionContext.getFormContext();
//Variable Declaration
//Environment Variable Schema Name Set In This Variable
var schemaName = "cc_CRMCrateServiceID";
//Check Get Environmental Variable Flag
var GetEnvVarFlag = formContext.getAttribute("cc_getenvironmentvariable").getValue();
if(GetEnvVarFlag == true){
//Start Progress Indicator
Xrm.Utility.showProgressIndicator("CRM Crate - Getting Environment Variable");
//Operation For Getting An Existing Value From An Environmental Variable
let GetEnvironmentVariableResult = await GetEnvironmentVariable(schemaName);
//Set Value In CRM Field
formContext.getAttribute("cc_environmentalvariablevalue").setValue(GetEnvironmentVariableResult);
//Stop Progress Indicator
Xrm.Utility.closeProgressIndicator();
}
}
//Main Method For Setting Variable
async function EnvironmentalVariableExecutionSet (executionContext) {
//Create a Form Context.
var formContext = executionContext.getFormContext();
//Variable Declaration
//Environment Variable Schema Name Set In This Variable
var schemaName = "cc_CRMCrateServiceID";
//Check Set Environmental Variable Flag
var SetEnvVarFlag = formContext.getAttribute("cc_setenvironmentvariable").getValue();
//We Will Capture Input From CRM Form & Use It As A New Value For Our Environment Variable
var SetEnvVarValue = formContext.getAttribute("cc_environmentvariablevalueset").getValue();
if(SetEnvVarFlag == true){
//Start Progress Indicator
Xrm.Utility.showProgressIndicator("CRM Crate - Setting Environment Variable");
//Operation For Getting An Existing Value From An Environmental Variable
await setEnvironmentVariable(schemaName, SetEnvVarValue);
//Stop Progress Indicator
Xrm.Utility.closeProgressIndicator();
}
}
//Function To Retrieve Environmental Variable
async function GetEnvironmentVariable(schemaName) {
var value = null;
var responseData = await Xrm.WebApi.retrieveMultipleRecords("environmentvariabledefinition", "?$top=1" +
"&$select=environmentvariabledefinitionid,defaultvalue" +
"&$expand=environmentvariabledefinition_environmentvariablevalue($select=value)" +
`&$filter=schemaname eq '${schemaName}'`);
if (responseData && responseData.entities && responseData.entities.length > 0) {
var responseDefinition = responseData.entities[0];
// Collecting Default Value From Variable
value = responseDefinition.defaultvalue;
// Get the related value if provided
if (responseDefinition.environmentvariabledefinition_environmentvariablevalue.length > 0) {
value = responseDefinition.environmentvariabledefinition_environmentvariablevalue[0].value;
}
}
return value;
}
//Function To Set Evironmental Variable
async function setEnvironmentVariable(EnvironmentSchemaName, inputValue, displayName) {
if (inputValue !== null && typeof inputValue !== "undefined") { inputValue = inputValue.toString(); }
// Retrieve Environmental Variable Data
var responseData = await Xrm.WebApi.retrieveMultipleRecords("environmentvariabledefinition", "?$top=1" +
"&$select=environmentvariabledefinitionid" +
"&$expand=environmentvariabledefinition_environmentvariablevalue($select=environmentvariablevalueid)" +
`&$filter=schemaname eq '${EnvironmentSchemaName}'`);
if (responseData && responseData.entities && responseData.entities.length > 0) {
var responseDefinition = responseData.entities[0];
var responseDefinationId = responseDefinition.environmentvariabledefinitionid;
if (responseDefinition.environmentvariabledefinition_environmentvariablevalue.length > 0) {
// Updating Related Record Value If It Exists
var valueId = responseDefinition.environmentvariabledefinition_environmentvariablevalue[0].environmentvariablevalueid;
await Xrm.WebApi.updateRecord("environmentvariablevalue", valueId, { "value": inputValue });
}
else {
// Creating Related Record Value If It Does Not Exists
var attributes = {
"value": inputValue,
"EnvironmentVariableDefinitionId@odata.bind": `/environmentvariabledefinitions(${responseDefinationId})`
};
await Xrm.WebApi.createRecord("environmentvariablevalue", attributes);
}
}
else {
// Definition doesn't exist - Deep create both definition and value
var attributes = {
"schemaname": EnvironmentSchemaName,
"displayname": displayName || key,
"environmentvariabledefinition_environmentvariablevalue": [
{
"value": inputValue
}
]
};
await Xrm.WebApi.createRecord("environmentvariabledefinition", attributes);
}
}
JavaScript snippet spefic to retrieving / getting an environment variable –
//Function To Retrieve Environmental Variable
async function GetEnvironmentVariable(schemaName) {
var value = null;
var responseData = await Xrm.WebApi.retrieveMultipleRecords("environmentvariabledefinition", "?$top=1" +
"&$select=environmentvariabledefinitionid,defaultvalue" +
"&$expand=environmentvariabledefinition_environmentvariablevalue($select=value)" +
`&$filter=schemaname eq '${schemaName}'`);
if (responseData && responseData.entities && responseData.entities.length > 0) {
var responseDefinition = responseData.entities[0];
// Collecting Default Value From Variable
value = responseDefinition.defaultvalue;
// Get the related value if provided
if (responseDefinition.environmentvariabledefinition_environmentvariablevalue.length > 0) {
value = responseDefinition.environmentvariabledefinition_environmentvariablevalue[0].value;
}
}
return value;
}
JavaScript snippet spefic to updating/ setting an environment variable –
//Function To Set Evironmental Variable
async function setEnvironmentVariable(EnvironmentSchemaName, inputValue, displayName) {
if (inputValue !== null && typeof inputValue !== "undefined") { inputValue = inputValue.toString(); }
// Retrieve Environmental Variable Data
var responseData = await Xrm.WebApi.retrieveMultipleRecords("environmentvariabledefinition", "?$top=1" +
"&$select=environmentvariabledefinitionid" +
"&$expand=environmentvariabledefinition_environmentvariablevalue($select=environmentvariablevalueid)" +
`&$filter=schemaname eq '${EnvironmentSchemaName}'`);
if (responseData && responseData.entities && responseData.entities.length > 0) {
var responseDefinition = responseData.entities[0];
var responseDefinationId = responseDefinition.environmentvariabledefinitionid;
if (responseDefinition.environmentvariabledefinition_environmentvariablevalue.length > 0) {
// Updating Related Record Value If It Exists
var valueId = responseDefinition.environmentvariabledefinition_environmentvariablevalue[0].environmentvariablevalueid;
await Xrm.WebApi.updateRecord("environmentvariablevalue", valueId, { "value": inputValue });
}
else {
// Creating Related Record Value If It Does Not Exists
var attributes = {
"value": inputValue,
"EnvironmentVariableDefinitionId@odata.bind": `/environmentvariabledefinitions(${responseDefinationId})`
};
await Xrm.WebApi.createRecord("environmentvariablevalue", attributes);
}
}
else {
// Definition doesn't exist - Deep create both definition and value
var attributes = {
"schemaname": EnvironmentSchemaName,
"displayname": displayName || key,
"environmentvariabledefinition_environmentvariablevalue": [
{
"value": inputValue
}
]
};
await Xrm.WebApi.createRecord("environmentvariabledefinition", attributes);
}
}
Validating the functionality in Dynamics 365 / Power Apps
Once the variable is created and the JavaScript snippet is deployed, navigate to the Dynamics 365 and verify the implementation of getting & setting values in an environmental variable as shown below.

Thus, we learned to get & set value in an Environment Variable of Dynamics 365 / Power Apps.