In this blogpost, we will learn to verify User level & Table level access / privileges in Power App using SDK for .NET (Plug-ins). Before we begin, ensure you subscribe to CRM Crate to stay updated in the field of Power Platform.
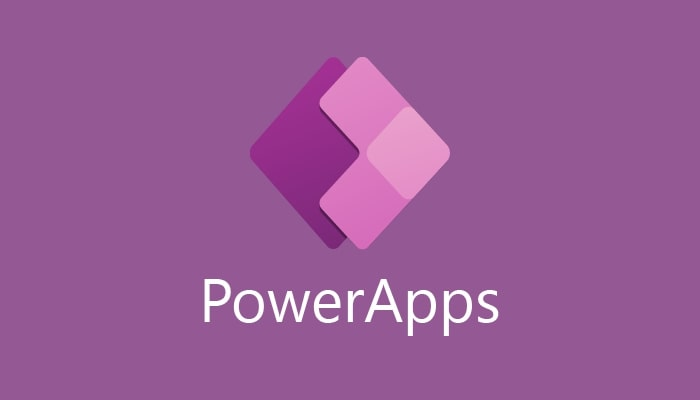
Power Apps provides a comprehensive range of tools for handling user-level privileges and permissions within the business applications. Below, we’ll deep dive into some important ideas and functionalities concerning user privileges and permissions in Power Apps:
- User Permissions: Permissions are like rules that determine what users are allowed to do in the app. You can decide who can create, view, edit, share, or delete stuff within the app.
- Security Roles: These come from the database you’re using with Power Apps. With security roles you can set up who can access what in the database, like tables and records.
- Sharing: When you share your app, you can choose who gets to use it and what they’re allowed to do with it. You can give some people just a look, while others can make changes.
- Environment Permissions: Power Apps lets you organize your apps and data into different environments. You can control who can access each environment and what they can do there.
- Data Permissions: You can set up rules to control who can see or change different parts of the data in your app. This helps keep sensitive info safe. One of the example of this is the “Field Security Profiles” in Model-Driven App.
Why do we need to check user permissions in Power Apps?
Below are few key reasons or checking user privileges in Power Apps:
- Keeping Things Safe / Security: We want to make sure only the right people can access sensitive stuff in our apps. Checking user privileges helps keep out anyone who shouldn’t be poking around where they don’t belong.
- Following the Rules / Compliance: There are laws and regulations about who can see what kind of data. By checking user privileges, we make sure we’re following those rules, whether it’s about personal info, medical records, or financial data.
- Protecting Our Data / Data Integrity: We need to make sure our data stays accurate and trustworthy. By controlling who can change or delete stuff, we keep our information in good shape for making decisions.
- Using Resources Wisely / Resource Management: We don’t want anyone hogging up resources they don’t need. By giving people only the access they need, we make sure our apps run smoothly without wasting resources.
- Making It Fit for Everyone / Personalization: Different people need different things from our apps. By checking user privileges, we can make sure each person sees what’s most useful to them and doesn’t get bogged down by stuff they don’t need.
Verifying User & Table Privileges in Power App using SDK for .NET (Plug-ins)
If you’re working on a synchronous plug-in, it’s crucial to remember that any data operation you try must be handled properly. If something goes wrong during such an operation, the entire data transaction will be revert as per the RollBack process. So, if there’s a part of your process that’s optional and depends on the user’s privileges, it’s a good idea to check those privileges before attempting the operation. This ensures smoother handling of exceptions and prevents unintended rollbacks.
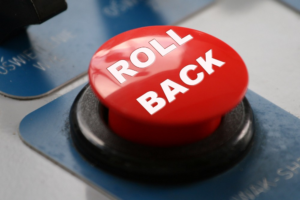
You have two approaches available to identify the operations a user can carry out:
- Verify individual table rows
- Verify the user’s security privileges
Verifying individual table rows
When users start interacting with specific table records, they typically begin with a query. If a user doesn’t have access to any records in that table, the query won’t return any results. In this case, the user’s options are limited to creating a new record. If an invoked user manages to fetch table records using a query, then you can follow the next step to check whether an user can assess a record by using the RetrievePrincipalAccess message.
Use the below method “GetAccessRights” for retrieving set of access rights for an user or team has for a table row.
static AccessRights GetAccessRights( IOrganizationService service, EntityReference userOrTeamOrOrganization, EntityReference entity) { var request = new RetrievePrincipalAccessRequest() { Principal = userOrTeamOrOrganization, //Entity Reference object of your invoked user / team / organization Target = entity //Logical name of your table }; var response = (RetrievePrincipalAccessResponse)service.Execute(request); return response.AccessRights; //Return Object }
The following code block demonstrates how to use the GetAccessRights method to verify whether an invoked user has access to append records to a table named “crmcrate_vendor” record using the AppendToAccess member.
var whoIAm = (WhoAmIResponse)service.Execute(new WhoAmIRequest()); var userObject = new EntityReference("systemuser", whoIAm.UserId); QueryExpression query = new("crmcrate_vendor") { ColumnSet = new ColumnSet("crmcrate_name"), TopCount = 1 }; EntityCollection ecVendors = service.RetrieveMultiple(query); EntityReference vendor = ecVendors .Entities .FirstOrDefault() .ToEntityReference(); AccessRights rights = GetAccessRights(service, userObject, vendor); var verifyAppendTo = rights.HasFlag(AccessRights.AppendToAccess);
Verify the user’s security privileges
When you’re not dealing with a dedicated table record to test, like when you’re verifying if a system user can create a new row in a table, you’ll need to depend on checking the user’s security privileges. These privileges are kept in the Privilege table of Dataverse.
In the Dataverse database, there are nearly 1000 unique security privileges, and this number keeps increasing as more tables are added to the system. To get a list of the privileges available in your environment, you can execute the following FetchXML query.
<fetch version='1.0' distinct='true' no-lock='true' > <entity name='privilege' > <attribute name='name' /> </entity> </fetch>
The value of the name field / attribute is used to store the name of privilege which follows this naming convention pattern when the privilege applies to tables in the format as “prv+Verb+Table SchemaName”. The verb can hold the values such as “Append, AppendTo, Assign, Create, Delete, Share, Write“.
Below are the messages supported by Privilege table.
Message | Web API Operation | SDK class or method |
---|---|---|
Retrieve | GET /privileges(privilegeid) See Retrieve | RetrieveRequest or Retrieve |
RetrieveMultiple | GET /privileges See Query Data | RetrieveMultipleRequest or RetrieveMultiple |
Below are the permission properties supported by Privilege table. Note: These properties are stored in the form of attributes in the Privilege table.
AccessRight
Rights a user has to an instance of an entity.
CanBeBasic
Information that specifies whether the privilege applies to the user, the user’s team, or objects shared by the user.
CanBeDeep
Information that specifies whether the privilege applies to child business units of the business unit associated with the user.
CanBeEntityReference
Information that specifies whether the privilege applies to the local reference of an external party.
CanBeGlobal
Information that specifies whether the privilege applies to the entire organization.
CanBeLocal
Information that specifies whether the privilege applies to the user’s business unit.
CanBeParentEntityReference
Information that specifies whether the privilege applies to parent reference of the external party.
CanBeRecordFilter
Information that specifies whether the privilege applies to the record filters.
The below table shows the sample content of Privilege table in Dataverse.
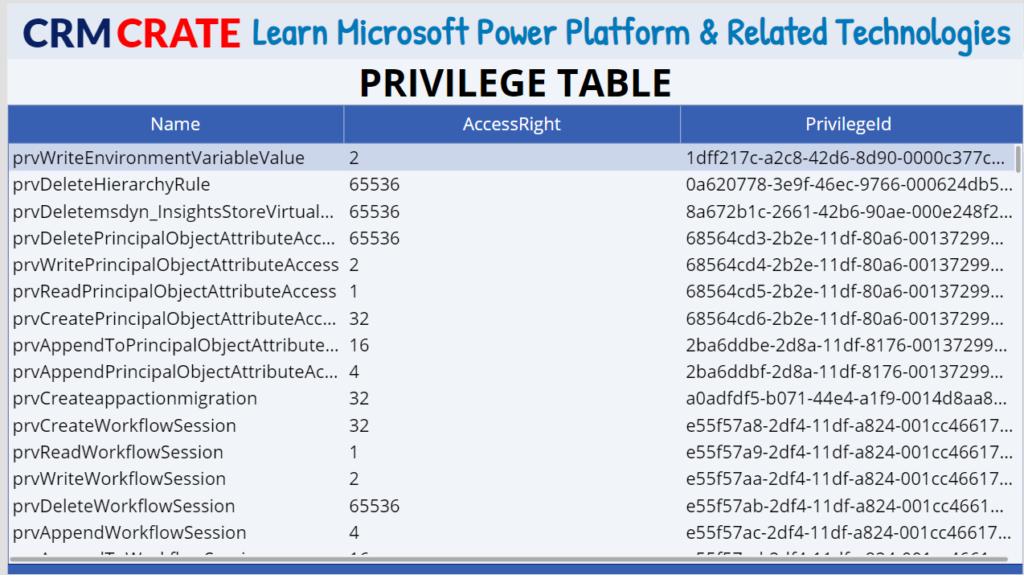
Here is an example that demonstrate how to use the RetrieveUserPrivilegeByPrivilegeName message. This message retrieves the list of privileges for an user from all direct roles linked to that user and from all indirect roles tied to teams in which the user is a member, based on the specified privilege name.
The following HasPrivilege static method returns whether the user has the named privilege.
static bool HasPrivilege(IOrganizationService service, Guid systemUserID, string privilegeName) { var request_Privilege = new RetrieveUserPrivilegeByPrivilegeNameRequest { PrivilegeName = privilegeName, UserId = systemUserID }; var response_Privilege = (RetrieveUserPrivilegeByPrivilegeNameResponse)service .Execute(request_Privilege ); if (response_Privilege .RolePrivileges.Length > 0) { return true; //User has the given privilege } return false; //User does not have the given privilege }
I came across this awesome site a couple days ago, they publish fantastic content for readers. The site owner knows how to educate customers. I’m pleased and hope they keep up the good work!
Thank you for your feedback.