We will learn to create a new PowerShell project and connect to the Microsoft Dynamics CRM using the PowerShell script. Before we start CLICK HERE to understand basics of PowerShell and need for using it in Microsoft CRM operations and automations. So lets start creating our new PowerShell project.
Download essential CRM assemblies.
As discussed earlier, the PowerShell operates same as that of a C# console application. Therefore we need the core Microsoft CRM assemblies to connect and perform operation in CRM using the PowerShell. Download the assemblies named “Microsoft.Crm.Sdk.Proxy.dll, Microsoft.IdentityModel.Client.ActiveDirectory.dll, Microsoft..IdentityModel.dll and Microsoft.Xrm.Sdk.dll”
- Download – Microsoft.Crm.Sdk.Proxy.dll
- Download – Microsoft.IdentityModel.Client.ActiveDirectory.dll
- Download – Microsoft..IdentityModel.dll
- Download – Microsoft.Xrm.Sdk.dll
Create a project location.
Once you have downloaded the nugget assemblies, now it is time to create a folder which will store your project. Create a local folder in your Computer and paste the above download assemblies in it.
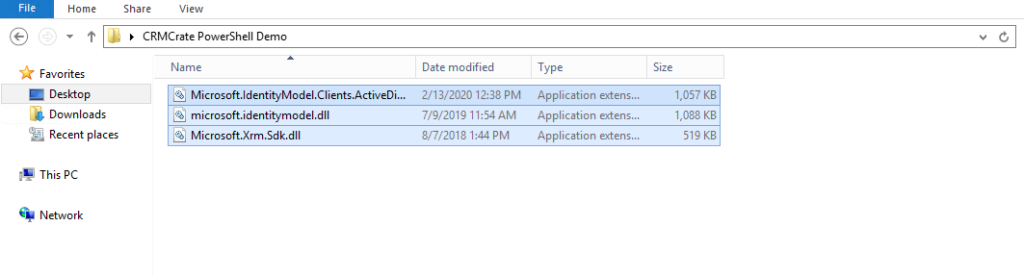
Create a new .ps1 extension file.
The PowerShell scripts supports files with extension (.ps1), (.psd1) or (.psm1). These file types are syntax colored in the Script Pane editor. To create a new script file, simply open a simple text file using a windows Text Document and save it as “YourFileName.ps1”.


Now in the Windows search box, search for “PowerShell ISE” and open it. Now the PoweShell ISE editor should get opened. Here, click on New >> Open and select our above created .ps1 file. So now your file should get completely opened in the editor as shown below.

PowerShell Script for connecting CRM.
We will first initialize our .NET and Microsoft assemblies by providing the path which is shown in below snippet. Note: – The variables in Powershell should start with “$” for example $VariableName.
#Initializing Assemblies. $scriptDir = Split-Path $script:MyInvocation.MyCommand.Path Write-Host "Current script directory is $scriptDir" Add-Type -Path "$scriptDir\Microsoft.IdentityModel.Clients.ActiveDirectory.dll"; Add-Type -Path "$scriptDir\Microsoft.Xrm.Sdk.dll" Add-Type -Path ([Reflection.Assembly]::LoadWithPartialName("System.IO")).Location; Add-Type -Path ([Reflection.Assembly]::LoadWithPartialName("System.Net")).Location; Add-Type -Path ([Reflection.Assembly]::LoadWithPartialName("System.Threading.Tasks")).Location; Add-Type -Path ([Reflection.Assembly]::LoadWithPartialName("System.Security")).Location; Add-Type -Path ([Reflection.Assembly]::LoadWithPartialName("System.Text.Encoding")).Location;
We have first initialized our variable which denotes the command script directories. Later we have given path of the .NET and Microsoft assemblies.
Now we will create the variables which stores the CRM instance url, Client ID and Client Secret.
#Initializing Configuration. $crmInstance = "https://crmcrate.crm.dynamics.com"; $crmClientId = "YOUR CRM CLIENT ID"; $crmClientSecret = "YOUR CRM SECRET ID";
Now we will create a S2S service which will return the service from which we will connect to the CRM.
#Initiliazing CRM S2S Service Proxy. function GetS2SService() { [System.Net.ServicePointManager]::SecurityProtocol = [System.Net.SecurityProtocolType]::Tls12; $clientcred = New-Object Microsoft.IdentityModel.Clients.ActiveDirectory.ClientCredential -ArgumentList ($CrmClientId, $CrmClientSecret); $authenticationContext = New-Object Microsoft.IdentityModel.Clients.ActiveDirectory.AuthenticationContext -ArgumentList ("https://login.microsoftonline.com/asd24tt-edr7-48af-8873-231f66d0bt560c"); $authenticationContext.TokenCache.Clear(); $authenticationResult = $authenticationContext.AcquireTokenAsync($CrmInstance, $clientcred).Result; $urlXRMSDK = New-Object System.Uri -ArgumentList ("$CrmInstance/xrmservices/2011/organization.svc/web?SdkClientVersion=8.2"); $_service = New-Object Microsoft.Xrm.Sdk.WebServiceClient.OrganizationWebProxyClient -ArgumentList ($urlXRMSDK, [System.TimeSpan]::MaxValue , $false); $_service.HeaderToken = $authenticationResult.AccessToken; return $_service; }
Now since we have the function which will return us the CRM service, lets perform the final step where we will call the above service. Below is the complete PowerShell script which will return the CRM service and connect your script with CRM.
#Initializing Assemblies. $scriptDir = Split-Path $script:MyInvocation.MyCommand.Path Write-Host "Current script directory is $scriptDir" Add-Type -Path "$scriptDir\Microsoft.IdentityModel.Clients.ActiveDirectory.dll"; Add-Type -Path "$scriptDir\Microsoft.Xrm.Sdk.dll" Add-Type -Path ([Reflection.Assembly]::LoadWithPartialName("System.IO")).Location; Add-Type -Path ([Reflection.Assembly]::LoadWithPartialName("System.Net")).Location; Add-Type -Path ([Reflection.Assembly]::LoadWithPartialName("System.Threading.Tasks")).Location; Add-Type -Path ([Reflection.Assembly]::LoadWithPartialName("System.Security")).Location; Add-Type -Path ([Reflection.Assembly]::LoadWithPartialName("System.Text.Encoding")).Location; Add-Type -Path ([Reflection.Assembly]::LoadWithPartialName("System.Text.Encoding")).Location; #Initializing Configuration. $crmInstance = "https://crmcrate.crm.dynamics.com"; $crmClientId = "YOUR CRM CLIENT ID"; $crmClientSecret = "YOUR CRM SECRET ID"; #Initiliazing CRM S2S Service Proxy. function GetS2SService() { [System.Net.ServicePointManager]::SecurityProtocol = [System.Net.SecurityProtocolType]::Tls12; $clientcred = New-Object Microsoft.IdentityModel.Clients.ActiveDirectory.ClientCredential -ArgumentList ($CrmClientId, $CrmClientSecret); $authenticationContext = New-Object Microsoft.IdentityModel.Clients.ActiveDirectory.AuthenticationContext -ArgumentList ("https://login.microsoftonline.com/asd24tt-edr7-48af-8873-231f66d0bt560c"); $authenticationContext.TokenCache.Clear(); $authenticationResult = $authenticationContext.AcquireTokenAsync($CrmInstance, $clientcred).Result; $urlXRMSDK = New-Object System.Uri -ArgumentList ("$CrmInstance/xrmservices/2011/organization.svc/web?SdkClientVersion=8.2"); $_service = New-Object Microsoft.Xrm.Sdk.WebServiceClient.OrganizationWebProxyClient -ArgumentList ($urlXRMSDK, [System.TimeSpan]::MaxValue , $false); $_service.HeaderToken = $authenticationResult.AccessToken; return $_service; } #Initializing Web Proxy. function GetS2SServiceOrgWebProxy() { $svc = $null; Write-Host "Connecting to CRM $CrmInstance"; $svc = GetS2SService; return $svc; } #Main Method. function Main (){ Write-Host "Connecting to the CRM $CrmInstance "; try{ $svc = GetS2SServiceOrgWebProxy; #YOU WILL RECEIVE THE MAIN SERVICE IN THIS VARIABLE. } catch { Write-Host "$_.Message"; } } #Call Main Method. Main;
The PowerShell script will initiate the operation with function “Main”. Further, as mentioned in the above code you will get the CRM service in the variable “$svc” which states your connection to the CRM. By using this service present in variable $svc, you can perform CRUD (Create – Read – Update – Delete) operation in CRM like any other .NET console application.
To run the PoweShell script, simply navigate to the top panel bar and click on run button as shown below.

Do subscribe us if this content helped you!
CRM Crate
All In One Platform For Learning Microsoft CRM.
[…] How to connect to CRM using PowerShell? […]