
We will learn how to read or retrieve, update, create and delete record in Microsoft Dynamics CRM using plugins. The plugins are generally used to drive the complex functionality in CRM, so there will be definitely some cases where we have to perform CRUD operation for various entity records. Before we start, make sure you have gone through the below links to understand all core concepts of plugins.
- What is a plugin?
- Event execution pipeline in plugin
- Developing & registering a plugin
- Reading & writing in fields using a plugin
- Retrieving records using a plugin
- Understanding images in plugin
- Difference between synchronous & asynchronous plugins
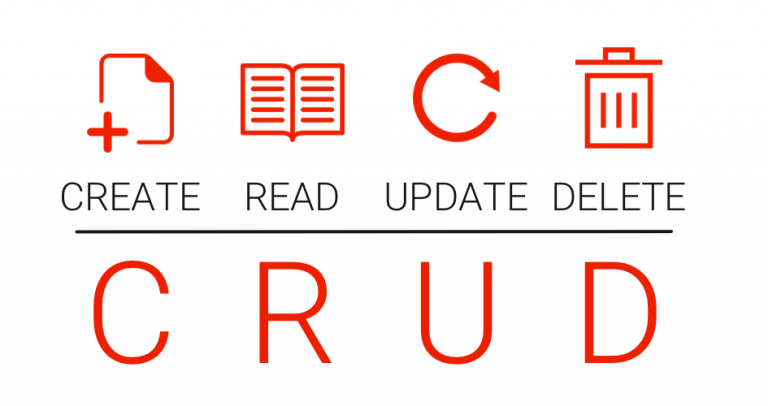
Reading values from record using a plugin
Scenario –
We will retrieve different field values of Account entity after creation of Account record.
Code –
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using Microsoft.Xrm.Sdk; using Microsoft.Xrm.Sdk.Query; namespace CRM_Crate___My_Sample_Plugin { public class Plugin : IPlugin { //Main Execute Method. public void Execute(IServiceProvider serviceProvider) { //Initializing Service Context. IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext)); IOrganizationServiceFactory factory = (IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory)); IOrganizationService service = factory.CreateOrganizationService(context.UserId); try { //Defining Entity Object. Entity eTarget = null; if (context.MessageName == "Create") { //Stage 1 - Retrieving Target Entity. eTarget = (context.InputParameters.Contains("Target") && context.InputParameters["Target"] != null) ? context.InputParameters["Target"] as Entity : null; //Stage 2 - Read Record Scenario. string AccountName = eTarget.GetAttributeValue("accountname"); int AccountNumber = eTarget.GetAttributeValue ("accountnumber"); EntityReference ContactLookup = eTarget.GetAttributeValue ("contact"); DateTime AccountDate = eTarget.GetAttributeValue ("accountdate"); Money AccountRevenue = eTarget.GetAttributeValue ("accountrevenue"); int AccountOptionset = eTarget.GetAttributeValue ("accountrevenue").Value; } } catch (Exception ex) { throw new InvalidPluginExecutionException(ex.Message); } } } }
Explanation –
- Stage 1 : Reading target account entity from input parameters.
- Stage 2 : Retrieve values from various fields with different data types and storing them in the given variables.
Updating a record using a plugin
Scenario –
We will update Contact entity and insert value in Contact’s field “Contact Name” on creation of Account record if account number equals “100”,
Code –
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using Microsoft.Xrm.Sdk; using Microsoft.Xrm.Sdk.Query; namespace CRM_Crate___My_Sample_Plugin { public class Plugin : IPlugin { //Main Execute Method. public void Execute(IServiceProvider serviceProvider) { //Initializing Service Context. IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext)); IOrganizationServiceFactory factory = (IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory)); IOrganizationService service = factory.CreateOrganizationService(context.UserId); try { //Defining Entity Object. Entity eTarget = null; if (context.MessageName == "Create") { //Stage 1 - Retrieving Target Entity. eTarget = (context.InputParameters.Contains("Target") && context.InputParameters["Target"] != null) ? context.InputParameters["Target"] as Entity : null; //Stage 2 - Read Record Scenario. string AccountName = eTarget.GetAttributeValue("accountname"); int AccountNumber = eTarget.GetAttributeValue ("accountnumber"); EntityReference ContactLookup = eTarget.GetAttributeValue ("contact"); DateTime AccountDate = eTarget.GetAttributeValue ("accountdate"); Money AccountRevenue = eTarget.GetAttributeValue ("accountrevenue"); int AccountOptionset = eTarget.GetAttributeValue ("accountrevenue").Value; //Stage 3 - Creating Query Expression. QueryExpression qeContactUpdate = new QueryExpression("contact"); qeContactUpdate.ColumnSet.AddColumns("contactnumber", "contactname", "contactid"); FilterExpression filterUpdate = new FilterExpression(LogicalOperator.And); filterUpdate.AddCondition("contactnumber", ConditionOperator.Equal, AccountNumber); qeContactUpdate.Criteria.AddFilter(filterUpdate); EntityCollection entityCollectionUpdate = service.RetrieveMultiple(qeContactUpdate); if (entityCollectionUpdate.Entities.Count == 0) { // No Contact Found. } else { //Stage 4 - Fetched Entity Object Record. foreach (var entity in entityCollectionUpdate.Entities) { Entity eContactUpdate = new Entity("contact"); //Stage 5 - Mapping Entity Id eContactUpdate.Id = entity.Id; eContactUpdate["contactname"] = "Test 1234"; //Stage 6 - Generating Update Request. service.Update(eContactUpdate); } } } } catch (Exception ex) { throw new InvalidPluginExecutionException(ex.Message); } } } }
Explanation –
- Stage 1 : Reading target account entity from input parameters.
- Stage 2 : Retrieve values from various fields with different data types and storing them in the given variables.
- Stage 3 : Creating query expression to retrieve contact record which is having same value in fields “Account Number” as that of Account.
- Stage 4 : Assigning retrieved entity from entity collection to the new entity object.
- Stage 5 : In order to send the update request, we will need to assign the record id of the Contact record which is to be updated in the newly created entity object “eContactUpdateId”. Later, assigning value “Test 1234” in Contact’s field “contactname”.
- Stage 6 : Once our entity object is ready, we will send this entity object in update request. This will update the same Contact record with new value in field Contact Name.
Creating a record using a plugin
Scenario –
Here, we will create a new Contact record on creation of Account record if the field “Account Number” is having value 100.
Code –
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using Microsoft.Xrm.Sdk; using Microsoft.Xrm.Sdk.Query; namespace CRM_Crate___My_Sample_Plugin { public class Plugin : IPlugin { //Main Execute Method. public void Execute(IServiceProvider serviceProvider) { //Initializing Service Context. IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext)); IOrganizationServiceFactory factory = (IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory)); IOrganizationService service = factory.CreateOrganizationService(context.UserId); try { //Defining Entity Object. Entity eTarget = null; if (context.MessageName == "Create") { //Stage 1 - Retrieving Target Entity. eTarget = (context.InputParameters.Contains("Target") && context.InputParameters["Target"] != null) ? context.InputParameters["Target"] as Entity : null; //Stage 2 - Read Record Scenario. string AccountName = eTarget.GetAttributeValue("accountname"); int AccountNumber = eTarget.GetAttributeValue ("accountnumber"); EntityReference ContactLookup = eTarget.GetAttributeValue ("contact"); DateTime AccountDate = eTarget.GetAttributeValue ("accountdate"); Money AccountRevenue = eTarget.GetAttributeValue ("accountrevenue"); int AccountOptionset = eTarget.GetAttributeValue ("accountrevenue").Value; if (AccountNumber == 100) { //Stage 3 - Creating New Entity Object Entity eNewContact = new Entity("contact"); eNewContact["ContactNumber"] = AccountNumber; //Stage 4 - Send Create Request. service.Create(eNewContact); } } } catch (Exception ex) { throw new InvalidPluginExecutionException(ex.Message); } } } }
Explanation –
- Stage 1 : Reading target account entity from input parameters.
- Stage 2 : Retrieve values from various fields with different data types and storing them in the given variables.
- Stage 3 : We have created a new entity object of Contact entity. Later, we have assigned Account Number value in field of Contact’s field “Contact Number”.
- Stage 4 : Once our entity object is ready, we will send this entity object in Create request. This will Create the Contact record with value in field Contact Number as account number.
Deleting a record using a plugin
Scenario –
Here, we have deleted the Contact record as soon as a new Account record is created when the value of field “Account Number” matches in both Contact and Account entity.
Code –
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using Microsoft.Xrm.Sdk; using Microsoft.Xrm.Sdk.Query; namespace CRM_Crate___My_Sample_Plugin { public class Plugin : IPlugin { //Main Execute Method. public void Execute(IServiceProvider serviceProvider) { //Initializing Service Context. IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext)); IOrganizationServiceFactory factory = (IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory)); IOrganizationService service = factory.CreateOrganizationService(context.UserId); try { //Defining Entity Object. Entity eTarget = null; if (context.MessageName == "Create") { //Stage 1 - Retrieving Target Entity. eTarget = (context.InputParameters.Contains("Target") && context.InputParameters["Target"] != null) ? context.InputParameters["Target"] as Entity : null; //Stage 2 - Read Record Scenario. string AccountName = eTarget.GetAttributeValue("accountname"); int AccountNumber = eTarget.GetAttributeValue ("accountnumber"); EntityReference ContactLookup = eTarget.GetAttributeValue ("contact"); DateTime AccountDate = eTarget.GetAttributeValue ("accountdate"); Money AccountRevenue = eTarget.GetAttributeValue ("accountrevenue"); int AccountOptionset = eTarget.GetAttributeValue ("accountrevenue").Value; //Stage 3 - Creating Query Expression. QueryExpression qeContactDelete = new QueryExpression("contact"); qeContactDelete.ColumnSet.AddColumns("contactnumber", "contactname", "contactid"); FilterExpression filterDelete = new FilterExpression(LogicalOperator.And); filterDelete.AddCondition("contactnumber", ConditionOperator.Equal, AccountNumber); qeContactDelete.Criteria.AddFilter(filterDelete); EntityCollection entityCollectionDelete = service.RetrieveMultiple(qeContactDelete); if (entityCollectionDelete.Entities.Count == 0) { // No Contact Found. } else { //Stage 4 - Fetched Entity Object Record. foreach (var entity in entityCollectionDelete.Entities) { Entity eContactDelete = new Entity("contact"); //Stage 5 - Mapping Entity Id eContactDelete.Id = entity.Id; //Stage 6 - Generating Update Request. service.Delete("contact", eContactDelete.Id); } } } } catch (Exception ex) { throw new InvalidPluginExecutionException(ex.Message); } } } }
Explanation –
- Stage 1 : Reading target account entity from input parameters.
- Stage 2 : Retrieve values from various fields with different data types and storing them in the given variables.
- Stage 3 : Creating query expression to retrieve contact record which is having same value in fields “Account Number” as that of Account.
- Stage 4 : Assigning retrieved entity from entity collection to the new entity object.
- Stage 5 : Mapping Id of the above retrieved entity and assigning it to the new entity object of Contact record.
- Stage 6 : Since we have the schema name and GUID or record Id of the contact to be deleted, we have sent a delete request in format of service.Delete(Schema Name Of Entity To Be Delete, GUID Of Record To Be Deleted);
Thus, we learned how to Create, Read, Update and Delete (CRUD) records in Microsoft Dynamics CRM using plugins.
CRM Crate
All In One Platform For Learning Microsoft CRM.