We will learn and understand Service Protection API limits in Power Apps / Dynamics 365. Before we start, make sure to subscribe to CRM Crate so that you can stay up to date in the field of Power Platform.
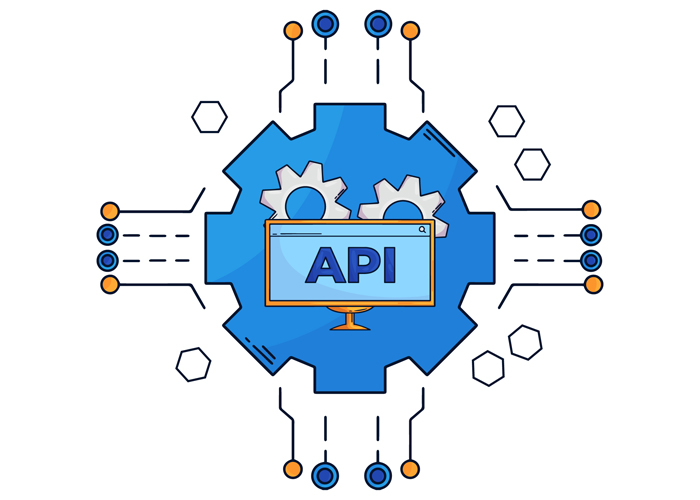
What are the new API limits in Power Apps?
In order to maintain consistent availability and performance for all users, certain restrictions are imposed on the usage of APIs. These restrictions have been put in place to identify instances where client applications are placing excessive demands on server resources.
These limitations should not impact regular users of interactive clients. They are specifically designed to have an effect only on client applications that make exceptionally high API requests. These limits serve as a protective measure against sudden and unexpected spikes in request volumes that could potentially disrupt the availability and performance of the Microsoft Dataverse platform.
When a client application makes requests that exceed the defined limits, the Dataverse platform follows a standard online service protocol by returning an error message indicating an excessive number of requests has been made.
Understanding impact of API limits on various client applications
Data integration applications
Applications intended for data loading or bulk updates within Dataverse need to have the capability to handle API limit errors related to service protection. These applications focus on maximizing data throughput to expedite their tasks. It is imperative for such applications to incorporate a well-defined strategy for retrying operations. Multiple strategies are available for them to employ in order to achieve the highest possible throughput.
Click on the given link to know more about maximizing throughput.
Interactive client applications
The service protection limits have been set at a level where it should be infrequent for an individual using an interactive client application to come across these limits under typical usage conditions. Nevertheless, this could occur when a client application permits bulk operations. Hence, developers of client applications should have an understanding of how service protection API limits are implemented and should devise user interfaces that minimize the likelihood of users sending exceptionally resource-intensive requests to the server. However, they should remain ready for the possibility of service protection API limit errors and have mechanisms in place to handle them.
It is important for client application developers not to simply throw the error and display the message to the user without further action or consideration.
Portal applications
Portal applications commonly direct requests from anonymous users via a service principal account. Given that service protection API limits are determined on a per-user basis, portal applications can potentially encounter these limits due to the volume of traffic they generate. Similar to interactive client applications, it is not anticipated that service protection API limit errors would be visible to the end users of the portal. Instead, the user interface should be designed to deactivate additional requests and present a message indicating that the server is currently occupied. This message may also specify the time when the application will resume accepting new requests.
Plug-ins and custom workflow activities
Plug-ins and custom workflow activities execute business logic in response to incoming requests. Unlike standard service protection limits, plug-ins and custom workflow activities are exempt from such limitations. They operate within an isolated sandbox service, where Dataverse operations are performed without utilizing public API endpoints.
If your application engages in operations that activate custom logic, the volume of requests initiated by plug-ins or custom workflow activities will not be factored into the service protection API limits. Nonetheless, the extra processing time incurred by these operations will be included in the total computation time associated with the initial request that triggered them. This computation time is considered part of the service protection API limits.
Using Retry operations for compensating API Limit
When a service protection API limit error occurs, it will provide a value indicating the duration before any new requests from the user can be processed. Below are the two different Retry operations supported by Microsoft.
- When a ‘429’ error is returned from the Web API while executing a request from client side, the response will include a ‘Retry-After’ with number of seconds included within the response.
- With the SDK for .NET, while executing a request from server side, a TimeSpan value is returned in the OrganizationServiceFault.ErrorDetails collection with the key
Retry-After
.
The Retry-After duration is contingent upon the complexity of the operations dispatched within the previous 5-minute timeframe. The more resource-intensive the requests, the extended the server’s recovery time will be. Today, because of the way the limits are evaluated, you can expect to exceed the number of requests and execution time limits for a 5 minute period before the service protection API limits will take effect. However, exceeding the number of concurrent requests will immediately return an error.
Implementing Retry After for SDK .NET –
Microsoft.Xrm.Tooling.Connector.CrmServiceClient nugget is recommended if you are using the SDK for .NET. Click here to more about the ‘Microsoft.Xrm.Tooling.Connector.CrmServiceClient ‘.
Since you will be using Xrm.Tooling.Connector, it will automatically pause and re-send the request after the Retry-After duration period.
Implementing Retry After for Web API-
If you’re utilizing the Web API in conjunction with a client library, you might discover that the library is designed to adhere to the expected retry behavior for 429 errors. If you’ve developed your own library, you have the option to incorporate behaviors similar to those found in this sample code for a helper WebAPIService class library in C#.
/// <summary> /// CRM Crate - Specifies the Retry policies /// </summary> /// <param name="config">Configuration data for the service</param> /// <returns></returns> static IAsyncPolicy<HttpResponseMessage> GetRetryPolicy(Config config) { return HttpPolicyExtensions .HandleTransientHttpError() .OrResult(httpResponseMessage => httpResponseMessage.StatusCode == System.Net.HttpStatusCode.TooManyRequests) .WaitAndRetryAsync( retryCount: config.MaxRetries, sleepDurationProvider: (count, response, context) => { int seconds; HttpResponseHeaders headers = response.Result.Headers; if (headers.Contains("Retry-After")) { seconds = int.Parse(headers.GetValues("Retry-After").FirstOrDefault()); } else { seconds = (int)Math.Pow(2, count); } return TimeSpan.FromSeconds(seconds); }, onRetryAsync: (_, _, _, _) => { return Task.CompletedTask; } ); }
Tracking remaining Retry Limit for WEB API
When making HTTP requests with the Web API, you have the ability to monitor the remaining limit values through the HTTP response headers provided.
Response Header | Value Description |
---|---|
x-ms-ratelimit-burst-remaining-xrm-requests | The remaining number of requests for this connection |
x-ms-ratelimit-time-remaining-xrm-requests | The remaining combined duration for all connections using the same user account |
It is not advisable to rely on these values to regulate the number of requests you send, as they are primarily meant for debugging purposes. If you decide to eliminate the affinity cookie, please be aware that these values will be reset when you connect to a different server.
Service Protection API Limit Errors
In this segment, we outline three categories of API limit errors related to service protection. Additionally, we delve into the factors contributing to these errors and explore potential strategies for addressing them.
Execution time error
This threshold monitors the cumulative execution time of incoming requests within the preceding 300-second timeframe. Certain operations consume more resources than others, such as batch operations, solution imports, and intricate queries, which can be particularly resource-intensive. Additionally, these operations may occur concurrently, allowing the potential for requesting tasks that collectively exceed 20 minutes of computational time within the 5-minute timeframe.
This limit may come into play when employing tactics that involve batch operations and simultaneous requests to circumvent the request quantity limit.
Error code | Hex code | Message |
---|---|---|
-2147015903 | 0x80072321 | Combined execution time of incoming requests exceeded limit of 1,200,000 milliseconds over time window of 300 seconds. Decrease number of concurrent requests or reduce the duration of requests and try again later. |
Number of requests error
This restriction tallies the cumulative volume of requests made within the preceding 5-minute interval. Under normal circumstances, an average user of an interactive application should not be able to surpass this limit by sending more than 1,200 requests per minute, unless the application grants users the ability to execute large-scale operations.
For instance, if a list view permits users to select 250 records at once and execute actions on all of them, a user would have to perform this action 24 times within a 5-minute window. In this scenario, each operation on the list must be completed within 12.5 seconds.
If your application offers this functionality, it’s advisable to contemplate the following approaches:
1. Combine the selected operations into a batch. A batch can contain up to 1000 operations and will avoid the number of requests limit. However, you will need to be prepared for the execution time limit.
2. Decreasing the total number of records that can be selected in a list. If the number of items displayed in a list is reduced to 50, the user would need to perform this operation 120 times within 300 seconds. The user would have to complete the operation on each list within 2.5 seconds.
Error code | Hex code | Message |
---|---|---|
-2147015902 | 0x80072322 | Number of requests exceeded the limit of 6000 over time window of 300 seconds. |
Concurrent requests error
This limit tracks the number of concurrent requests.
Client applications are not restricted to sending individual requests one after the other. Instead, clients have the flexibility to employ parallel programming patterns or various techniques to transmit multiple requests simultaneously. The server has the capability to identify situations where it is concurrently handling requests from the same user. If the number of simultaneous requests exceeds a certain threshold, an error will be triggered.
Sending concurrent requests can be a vital component of an approach to optimize throughput, but it’s essential to exercise caution and maintain control. When implementing Parallel Programming in .NET, the default level of parallelism is determined by the number of CPU cores available on the server executing the code. This level should not surpass the specified limit. You can set the ParallelOptions.MaxDegreeOfParallelism Property to establish an upper limit on the number of concurrent tasks.
Error code | Hex code | Message |
---|---|---|
-2147015898 | 0x80072326 | Number of concurrent requests exceeded the limit of 52. |
Thus, we learned the concept of Service API limits in Power Apps.