In this course, we will understand the concept of REST API and create a Twitter API using Python programming. Before we start, make sure to go through the below course for understanding core concepts of an API.
What is a REST API?
A REST API which is also known as RESTful API is an “application programming interface” that follows the protocol of REST architecture. The term “REST” stands for “Representational – Stateless – Transfer”. This API is a set of architectural principles, rather than a set of protocols or standards. API developers can implement REST in a variety of ways as per the technical or business needs. When a application (requester) makes a request via a RESTful API, it transfers a representation of the state of the resource to the requester. This information (or representation), is delivered in one of several formats via HTTP: JSON (Javascript Object Notation), HTML, XLT, or plain text. JSON is the most generally popular because, despite its name, it’s language agnostic, as well as readable by both humans and machines.
REST API Vs SOAP API
SOAP is another majorly used API which stands for Simple Object Access Protocol, a messaging standard defined by the W3C and its member editors. SOAP uses an XML data format to declare its request and response messages, relying on XML Schema and other technologies to enforce the structure of its payloads.
SOAP API | REST API |
SOAP stands for Simple Object Access Protocol. | REST stands for Representational State Transfer. |
SOAP is a protocol. | REST is an architectural style. |
SOAP uses services interfaces to expose the business logic. | REST uses URI to expose business logic. |
SOAP can’t use REST because it is a protocol. | REST can use SOAP web services because it is a concept and can use any protocol like HTTP, SOAP. |
JAX-WS is the java API for SOAP web services. | JAX-RS is the java API for RESTful web services. |
SOAP requires more bandwidth and resource than REST. | REST requires less bandwidth and resource than SOAP. |
SOAP defines standards to be strictly followed. | REST does not define too much standards like SOAP. |
SOAP defines its own security. | RESTful web services inherits security measures from the underlying transport. |
SOAP permits XML data format only. | REST permits different data format such as Plain text, HTML, XML, JSON etc. |
SOAP is less preferred than REST. | REST more preferred than SOAP. |
Create a Twitter application
We will create a Twitter application which will send request from your local machine to the Twitter application using fundamentals of REST API via the Python platform.
1. Create a Twitter developer account.
Navigate to the twitter developer website “https://developer.twitter.com/” and click on the button Apply.

Now, click on the button “Apply for a developer Account” as shown below. Once done, login with your existing twitter account credentials.

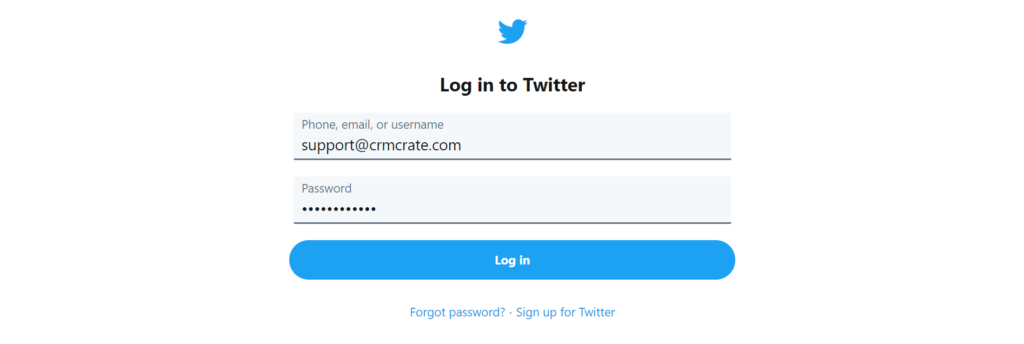
Submit the details which are been asked by the twitter and create your own Twitter developer account. Your developer account will look like the below shown image once your are completed with the necessary steps.
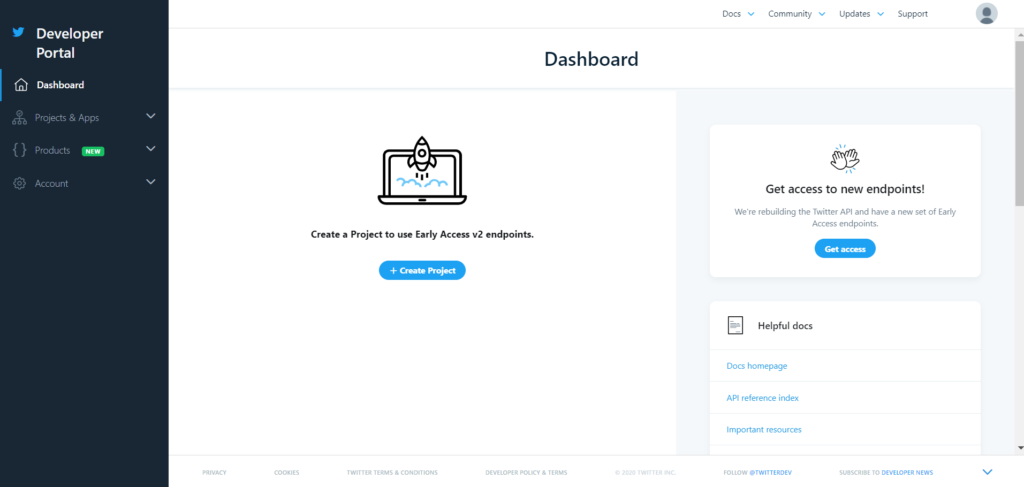
2. Create a Twitter Application.
Navigate to the above created Twitter developer account. Under Projects & Apps, click on Overview. This section will display all the existing projects and applications you have created in your developer account. In order to create a new application, navigate to the section called Standalone Apps and click on Create App.
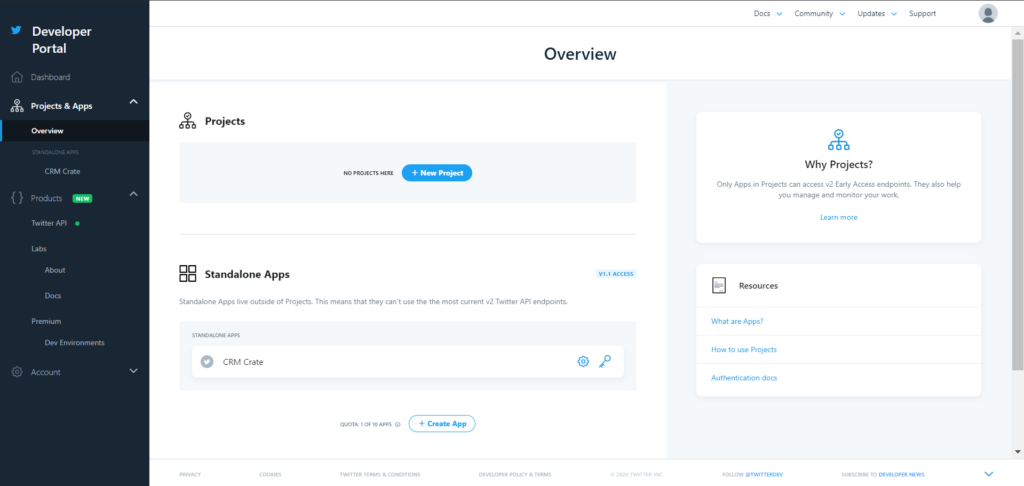
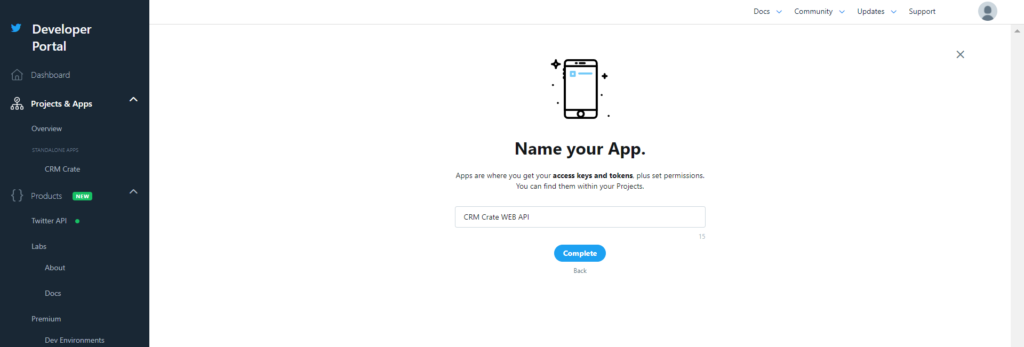
Once your application is created, the Twitter will provide you an API Key and API Secret as shown below. Copy these key and secret in your notepad. Further click on App Settings.

Here you will see the detailed configuration of your newly created application. Click on the App Permission and make sure your application has Read – Write access permssions as shown below.
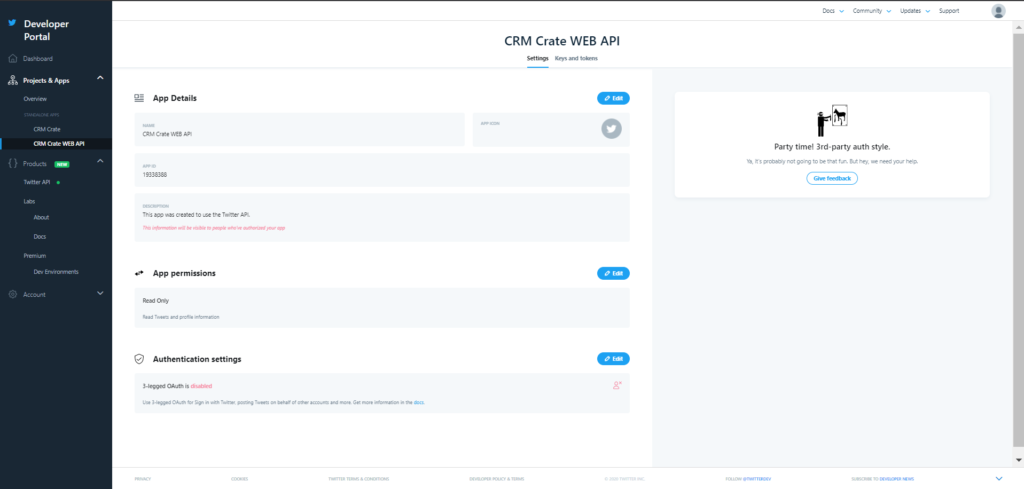

Again in the same window, open the Authentication Settings. Enable the 3-legged OAuth for signing into your twitter account. Provide the callback URL and your organizations website URL as shown below and click on save.


Now, inside your Twitter application, navigate to the section called “Keys & token”. Navigate to Access Token & Secret and click on generated. Copy the populated Access Token & Token Secret in your notepad.


3. Create a Python code for send a REST API request to your Twitter application.
We are now done with creating the Twitter application, now lets send a RESTful API request to our application using Python.
Click here to download and install the Python in your system.

Once you have downloaded the Python, create a folder in your local system and copy its path as shown below.
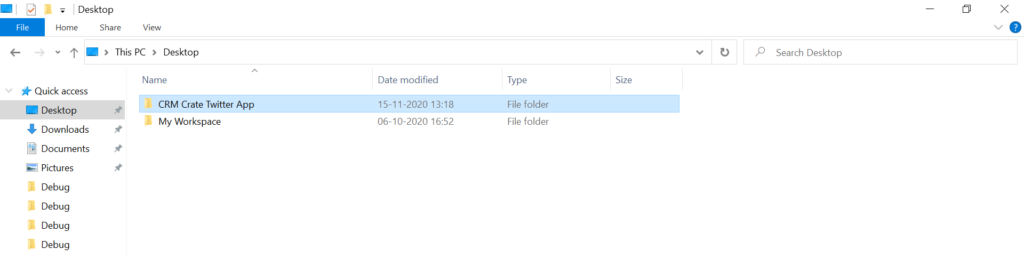
Note : – We will be using the Python – Twitter library called “Tweepy”. Click here to know more about the Tweepy library.
Open the Command Prompt Line and set its path to the above created folder.

Enter the below command in the CMD. This will create Tweepy libraries for supporting the Python program in your file.
pip install tweepy

Open the Notepad or Notepad ++. Copy the below code in it and save your file with extension as “.py” (Python File) in the above created folder.
import tweepy apiKey = 'Enter Your API Key' apiSecret = 'Enter Your API Secret' accessToken = 'Enter Your Access Token' accessTokenSecret = 'Enter Your Access Token Secret' def OAuth (): try: auth = tweepy.OAuthHandler(ak,aks) auth.set_access_token(at,ats) return auth except Exception as e: return none Oauth = OAuth() apicall = tweepy.API(Oauth) apicall.update_status('This is a sample tweet from CRM Crate System.') print('Tweet Created')

Code Explanation :
- Enter your API Key, API Secret, Access Token and Access Secret in the above defined variables.
- Initiate an object called “auth” with the above defined key and secret.
- Call the Tweepy API by passing the definition “OAuth”.
- Create an update RESTful API call with the text of your choice. This method will take the input given text and supply in via the HTTP body to your Twitter application.
- Print the success message once the tweet is created in the Twitter account.
Now, navigate back the the Command Prompt Line and execute the below code. Note : – Replace the “CRMCrate_TwitterAPI” with your file name.
python CRMCrate_TwitterAPI.py
Python will print the above defined success message after successfully executing the API as shown below.

Validate the API success in the Twitter profile
Lets check whether the above API request is successfully executed or not in the Twitter Handler. Open your regular Twitter account which is associated with the above created Twitter application. Here you can see the tweet is been successfully posted in your Twitter account with help of the RESTful API which we have implemented above.
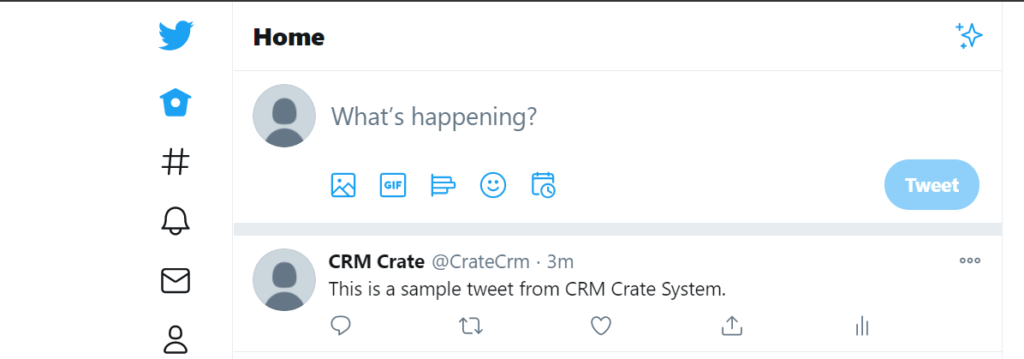
[…] What is REST API? | Create a Twitter API using Python […]